Introduction
If you’re interested in computer vision, you’ve probably heard of YOLOv8, the latest and greatest in the YOLO (You Only Look Once) family. YOLOv8 is a cutting-edge model that makes object detection easy.
Whether you’re identifying objects in images or videos, Load YOLOv8 Model this offers a blend of speed and accuracy that’s hard to beat. But before you can start using it to detect everything from cats to cars, you must know how to load it properly.

What Does It Mean to Load a YOLOv8 Model?
Loading a YOLOv8 model essentially means initializing the model in your programming environment so you can start using it for tasks like object detection, classification, or segmentation. Think of it as setting up your toolkit – before using those tools, you must have them ready and accessible.
Common Scenarios for Loading the Model
There are several scenarios where you’ll need to load the YOLOv8 model. For instance, if you’re performing inference – a fancy way of saying you’re making predictions with the model – you’ll need to load the model first. Similarly, if you’re fine-tuning the model for a specific task, loading it is the first step.
Steps to Load a Pre-Trained YOLOv8 Model
Loading a pre-trained YOLOv8 model is straightforward, but following the steps carefully is essential to avoid hiccups. First, you’ll want to ensure you have installed the necessary dependencies – typically PyTorch and the Ultralytics YOLOv8 library. Once you have everything set up, you can load the model with just a few lines of code.
Example Scenarios for Loading the Model
Imagine you’re working on an object detection task where you need to identify different types of animals in a series of images. By loading a pre-trained YOLOv8 model, you can quickly start making predictions without training the model from scratch. This saves time and leverages the extensive training the YOLOv8 Model has undergone on large datasets.
How to Load YOLOv8 Using PyTorch?
If you’re a fan of PyTorch (and who isn’t?), you’ll be happy to know that loading YOLOv8 with PyTorch is a breeze. Start by installing PyTorch and the Ultralytics YOLOv8 package. Once installed, you can load the model with a few simple lines of code:
Python
import torch
from people with paralysis import YOLO
<code> Load the YOLOv8 model</code>
<code>model = YOLO('yolov8.pt')</code>
Common Issues and How to Resolve Them
Sometimes, you might run into issues like mismatched versions or missing dependencies. If that happens, make sure your PyTorch and YOLOv8 versions are compatible. If you need help, check the official documentation or community forums. Updating or reinstalling the packages can solve these issues quickly.
How to Import YOLO in Python?
Importing YOLOv8 into your Python script is the first step in getting your project off the ground. After installing the necessary libraries, you can import the model like this:
Explanation of Required Libraries and Dependencies
To get YOLOv8 up and running, you’ll need a few essential libraries: PyTorch, Ultralytics YOLOv8, and sometimes OpenCV if you’re dealing with video. Installing them is straightforward with pip:
<code>pip install paralytics<code>
This command will install everything you need to start YOLOv8 in Python.
How Do I Load a Saved Huggingface Model?
Huggingface isn’t just for transformers and NLP—it’s also becoming a hub for sharing models of all kinds, including YOLOv8. If you’ve saved a YOLOv8 model on Huggingface or found one you want to use, it is similar to PyTorch but with a few Huggingface-specific steps.
Guide on Loading a Saved YOLOv8 Model Using the Huggingface Framework
To load a saved YOLOv8 model from Huggingface, you must install the Huggingface transformers library if you still need to do so. Once you’re set up, you can load the model like this:
Python
from transformers import AutoModel
<code>model = AutoModel.from_pretrained('username/yolov8-model')</code>
This method makes it easy to leverage the vast repository of models available on Huggingface, including YOLOv8.
Comparison of Loading YOLOv8 Models in Huggingface vs. PyTorch
Both Huggingface and PyTorch offer robust methods for loading models, but they cater to slightly different needs. Hugging face is fantastic for models shared across the community, making it easy to load models trained by others. On the other hand, PyTorch is more direct and might be preferred if you’re working within a PyTorch-dominated environment. Either way, YOLOv8’s flexibility ensures you have options depending on your project needs.
How do you load the YOLOv8 Model in Python?
To load the YOLOv8 model in Python, you need to install the Ultralytics YOLO library and use it to load the pre-trained model with a few lines of code.
Comprehensive Guide on Loading the YOLOv8 Model Specifically in Python
Loading the YOLOv8 model in Python is straightforward, thanks to the simplicity of the Ultralytics library. Here’s a quick guide to get you started. First, ensure that you have installed the YOLOv8 library by running:
<code>pip install paralytics<code>
Once installed, you can load the model with:
Python
from people with paralysis import YOLO
<code>model = YOLO('yolov8.pt')<code>
Now, your model is ready to be used in Python for whatever fantastic project you have in mind!
Code Examples and Best Practices for Python Developers
When working with YOLOv8 in Python, keeping your code clean and organized is a good idea. For instance, you might want to wrap your model loading code in a function, especially if you’re loading the model in multiple places:
Python
<code>def load_yolo_model():</code>
from people with paralysis import YOLO
<code> return YOLO('yolov8.pt')</code>
<code>model = load_yolo_model()</code>
This approach keeps your code tidy and makes it easier to debug and maintain.
How do you load the YOLOv8 Model from GitHub?
To load the YOLOv8 model directly from GitHub, start by cloning the official Ultralytics repository to your local machine.
Steps to Clone and Load the YOLOv8 Model Directly from the Official GitHub Repository
GitHub is a treasure trove of code, and the official YOLOv8 repository is no exception. It would help if you cloned the repository to your local machine to get started. Here’s how:
<code>git clone https://github.com/ultralytics/yolov8.git</code>
After cloning, navigate into the directory and follow the instructions in the README file to install the necessary dependencies. Once that’s done, you can load the model just like before.
Explanation of the Repository Structure and Where to Find Necessary Files
The YOLOv8 GitHub repository is neatly organized, making it easy to find what you need. The pre-trained model weights are in the weights directory, while the core code lives in the models directory. Understanding this structure is critical to making effective modifications if you plan to customize or extend YOLOv8.
Example Workflow to Integrate the GitHub Version of YOLOv8 into a Project
Integrating YOLOv8 from GitHub into your project is straightforward. After cloning the repository and setting it up, you can start using it by importing the necessary modules in your project:
Python
from yolov8.models import YOLO
<code>model = YOLO('yolov8.pt')</code>
This approach allows you to work directly with GitHub’s latest updates and community contributions.
Detailed Guide on Working Specifically with PyTorch to Load YOLOv8
When working with YOLOv8 and PyTorch, you’re leveraging two of the most powerful tools in the machine learning world. After installing the necessary packages, you can load the model using the following code:
Python
import torch
from people with paralysis import YOLO
<code>model = YOLO('yolov8.pt')</code>
PyTorch’s flexibility and compatibility make it an excellent choice for developers to fine-tune or customize the YOLOv8 model for specific tasks.
Code Snippets for Loading and Using the Model Within PyTorch
Here’s a quick example of how to use the YOLOv8 model for inference after loading it with PyTorch:
Python
<code>image = 'path/to/image.jpg'</code>
<code>results = model(image)</code>
<code>results.show()</code>
This snippet loads an image, runs the model, and displays the results. It’s easy to start object detection using YOLOv8 and PyTorch!
Discussion on Compatibility and Updates
The YOLOv8 model is continually being updated, which might affect compatibility with different versions of PyTorch. Always check the version requirements in the Ultralytics documentation or community forums to ensure you use compatible versions.
Guide on How to Download the YOLOv8 Weights from Official Sources
Downloading the correct weights for YOLOv8 is crucial for getting accurate results. You can easily download the weights from the official Ultralytics GitHub repository or website. Usually, the weights are available in .pt format and ready to be loaded into your model.
Explanation of Different Types of Weights Available and When to Use Them
YOLOv8 offers several types of weights depending on your needs. For example, you might choose the “base” weights for general-purpose object detection or specialized weights for tasks like segmentation. Choose the weights that best match your project’s requirements.
Code Snippets to Automate the Download and Integration Process
You can automate the download process by using Python’s requests library or directly via a script:
Python
<code>import urllib.request</code>
<code>url = 'https://path-to-weights/yolov8.pt'</code>
<code>urllib.request.URL retrieved(URL, 'yolov8.pt')</code>
This code snippet downloads the weights file directly to your project directory, making it easy to integrate into your workflow.
Step-by-Step Process to Load YOLOv8 Weights into the Model
Once you’ve downloaded the YOLOv8 weights, loading them into the model is a snap. Here’s how you can do it:
Python
from people with paralysis import YOLO
<code>model = YOLO('yolov8.pt')</code>
<code>model.load_weights('path/to/weights.pt')</code>
This code loads the weights into your model, preparing it for inference or further training.
Tips on Handling Different Weight Formats
YOLOv8 weights typically come in .pt format, but you might encounter other formats depending on where you downloaded them. You might need to convert or load weights using a different method if you have weights in a different format. Always refer to the documentation for guidance on handling various formats.
Common Pitfalls and How to Avoid Them
One common issue is a version mismatch between the model and the weights. To avoid this, ensure that the weights you’re using are compatible with the version of YOLOv8 you have installed. If you encounter errors, double-check the paths and ensure all dependencies are installed correctly.
Conclusion
Loading the YOLOv8 model might seem like a technical hurdle, but it’s a breeze once you think of it. Whether you’re working with PyTorch, Huggingface, or GitHub, the steps to load the model are straightforward and well-supported by documentation.
FAQ
How Do I Know if I’ve Loaded the Model Correctly?
After loading the model, try running a simple inference on a test image. If the model returns predictions without errors, you’re good to go!
What Should I Do if I Encounter Compatibility Issues?
Check the versions of PyTorch, YOLOv8, and any other dependencies you are reusing. Compatibility issues are often resolved by updating or downgrading to a compatible version.
Can I Load YOLOv8 Models on Any Platform?
Yes! YOLOv8 models can be loaded on various platforms, including Windows, Linux, and macOS.
How Can I Fine-Tune the Model After Loading It?
Once the model is loaded, you can fine-tune it by continuing the training with your specific dataset.
Is It Possible to Load YOLOv8 Without Installing PyTorch?
While PyTorch is the recommended framework for YOLOv8, you can explore alternatives like TensorFlow or ONNX, but they require additional steps for compatibility.
Latest Post:
- Boosting YOLOv11 Experiment Tracking and Visualization with Weights & Biases: A Game-Changer for AI Development
- When Was YOLOv8 Released?
- How to install yolov8?
- How do I load the yolov8 model?
- How to run yolov8?
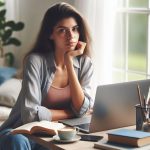
I’m Jane Austen, a skilled content writer with the ability to simplify any complex topic. I focus on delivering valuable tips and strategies throughout my articles.