Introduction
I’d be glad to assist you with crafting a comprehensive article on “How Can I Add Grad CAM YOLOv8?”. However, it’s essential to acknowledge that directly integrating Grad CAM to YOLOv8 core codebase isn’t currently supported by the official release.
Nevertheless, effective workarounds and alternative approaches exist to achieve similar functionality.
Here’s a detailed guide incorporating best practices and addressing potential challenges:

Understanding Grad-CAM and its Applicability to YOLOv8:
Grad-CAM (Gradient-weighted Class Activation Mapping) is a visualization technique that highlights image regions contributing most to a target class prediction in a CNN-based model. While originally designed for convolutional neural networks, it can be adapted to object detection models like YOLOv8 with careful considerations.
YOLOv8’s architecture differs from standard CNNs in terms of output structure and intermediate feature computation. This necessitates tailored strategies for incorporating Grad-CAM.
Approaches for Adding Grad CAM to YOLOv8
1: Leveraging External Grad-CAM Implementations:
- Explore external PyTorch-based Grad-CAM libraries like Grad-CAM or Torch Vision’s explainability module.
Adapt these libraries to YOLOv8’s unique structure, potentially involving:
- Extracting relevant intermediate features using hooks or custom layers.
- Modifying input/output handling to align with YOLOv8’s prediction format (bounding boxes, confidence scores).
- Carefully handling multiple predictions per image and class-specific visualization.
2: Custom Implementation based on Grad-CAM Principles:
For finer-grained control or integration into your specific workflow, consider a custom implementation:
- Implement the core Grad-CAM algorithm
- Backpropagate class activation gradients.
- Generate weighted feature maps using gradients and feature maps.
- Up sample weighted maps to input image size.
- Overlay or combine with the original image for visualization.
- Tailor the implementation to YOLOv8’s architecture and output format.
Key Considerations and Best Practices:
- Grad-CAM’s Limitations: Grad-CAM primarily reveals high-level discriminative regions and its interpretability can be subjective. Consider it as a qualitative tool rather than a definitive explanation.
- Addressing YOLOv8 Specificity: Remember that YOLOv8 uses multiple feature maps at different scales and outputs bounding boxes instead of class activations. Adapt Grad-CAM accordingly.
- Experimentation and Evaluation: Experiment with different Grad-CAM implementations or parameter settings to find what works best for your dataset and visualization goals. Evaluate the resulting visualizations to ensure they align with your understanding of the model’s behaviour.
By following these guidelines and carefully considering the provided approaches, you can effectively add Grad-CAM functionality to your YOLOv8 project, gaining valuable insights into your model’s decision-making process and improving its interpretability.
How Can I Add Grad Cam YOLOv8? Step by Step
Sure, adding Grad-CAM (Gradient-weighted Class Activation Mapping) to YOLOv8 involves several steps. Grad-CAM is a technique used for visualizing and understanding the decisions made by a neural network, particularly in convolutional neural networks (CNNs).
YOLOv8 (You Only Look Once version 8) is an object detection algorithm that can be enhanced with Grad-CAM to provide better insights into the model’s decision-making process.
Here’s a detailed guide on how to add Grad-CAM to YOLOv8:
1: Install the necessary libraries:
Make sure you have the required libraries installed. You can use the following commands to install them:
- bash
- pip install torch torchvision
- pip install numpy opencv-python
2: Clone the YOLOv8 repository:
Clone the YOLOv8 repository from GitHub using the following command:
- bash
- git clone https://github.com/ultralytics/yolov5.git
3: Install YOLOv8 dependencies:
Navigate to the YOLOv8 directory and install the dependencies:
- bash
- cd yolov5
- pip install -U -r requirements.txt
4: Modify the YOLOv8 code:
You’ll need to modify the YOLOv8 code to incorporate Grad-CAM. Open the YOLOv8 source code and locate the file models/yolo.py. In this file, you’ll find the YOLOv8 model architecture.
5: Add Grad-CAM module:
Add the Grad-CAM module to the YOLOv8 model architecture. You can use the torchvision library to import the Grad-CAM module. Insert the following lines of code after the import statements:
- python
- from torchvision import models
- class YOLOv8GradCAM(nn.Module):
- def __init__(self, base_model, feature_module, target_layer):
- super(YOLOv8GradCAM, self).__init__()
- self.model = base_model
- self.feature_module = feature_module
- self.target_layer = target_layer
- def forward(self, x):
- # Forward pass up to the target layer
- for layer_name, layer in self.model._modules[self.feature_module]._modules.items():
- x = layer(x)
- if layer_name == self.target_layer:
- break
- # Grad-CAM calculation
- feature_map = x
- _, _, H, W = feature_map.size()
- cam = F.relu(feature_map) # Apply ReLU to the feature map
- cam = F.adaptive_avg_pool2d(cam, 1) # Perform global average pooling
- cam = torch.mul(feature_map, cam) # Multiply the feature map by the CAM
- cam = cam.sum(dim=1, keepdim=True) # Sum along the channel dimension
- return cam
6: Integrate Grad-CAM with YOLOv8 predictions:
In the YOLOv8 code, after obtaining the model predictions, steps of fine-tuning YOLOv8 use use the Grad-CAM module to generate class activation maps. You can visualize these maps to better understand which regions of the input image contributed most to the model’s decision.
7: Visualize Grad-CAM output:
Use the generated Grad-CAM output to overlay heatmaps on the input images. This will highlight the regions that were crucial for the YOLOv8 model’s decision. You can use OpenCV or other image processing libraries for this visualization.
8: Test and evaluate:
After implementing Grad-CAM in YOLOv8, test the modified model on a set of images to ensure that Grad-CAM is working as expected. Evaluate the model’s performance and visualize the Grad-CAM results.
By following these steps, you should be able to integrate Grad-CAM with YOLOv8 and gain valuable insights into the model’s decision-making process. Remember to save the modified YOLOv8 code and Grad-CAM results for future reference and analysis.
Conclusion
Adding Grad-CAM to YOLOv8 enhances interpretability by providing insights into which regions of an image contribute most to the model’s predictions. This can be valuable for debugging and understanding model decisions.
The implementation involves modifying the YOLOv8 code to include Grad-CAM calculations and overlaying the generated heatmap on the original image during inference.
Grad-CAM is a powerful tool for visualizing the attention of deep neural networks and can be applied to various other computer vision models for similar insights.
FAQS (Frequently Asked Questions)
Q#1: What is Grad-CAM, and how does it enhance YOLOv8?
Grad-CAM (Gradient-weighted Class Activation Mapping) is a technique used for visualizing and understanding the decisions made by a neural network. When applied to YOLOv8, it highlights the regions of an image that contribute the most to the network’s predictions. This visualization aids in interpreting the model’s decision-making process and can be useful for debugging and improving model performance.
Q#2: How can I integrate Grad-CAM with YOLOv8?
Integrating Grad-CAM with YOLOv8 involves modifying the YOLOv8 code to include the necessary hooks and layers for computing the gradient-weighted activation maps. You’ll need to add the Grad-CAM functionality after the model has made predictions. Additionally, adapting visualization code to overlay the generated heatmaps onto the original images is crucial for effective interpretation.
Q#3: Are there any specific libraries or tools for implementing Grad-CAM with YOLOv8?
Yes, several Python libraries such as PyTorch and TensorFlow provide functionalities to implement Grad-CAM. You can leverage existing implementations and adapt them to work with the YOLOv8 architecture. Popular deep learning frameworks often have pre-built modules for Grad-CAM that you can use or customize for your specific YOLOv8 application.
Q#4: How does Grad-CAM help in object detection tasks using YOLOv8?
Grad-CAM helps in understanding where the YOLOv8 model is focusing its attention when making object predictions. By visualizing the regions of interest, you can gain insights into which parts of the input image contribute most significantly to the model’s decision. This information can be valuable for debugging, identifying false positives/negatives, and improving the overall accuracy of object detection.
Q#5: Can Grad-CAM be applied to different versions or custom variations of YOLO, or is it specific to YOLOv8?
Grad-CAM is a general technique applicable to various neural network architectures, and it is not specific to YOLOv8. You can implement Grad-CAM with different versions of YOLO or even with custom variations of the YOLO architecture. The key is to adapt the Grad-CAM implementation to the specific structure and layers of the YOLO model you are working with, ensuring compatibility and accurate visualization of feature importance.
Recent Posts
- YOLOv8 Aimbot: Challenges and Opportunities
- YOLOv8 Train Custom Dataset: Train Your Own Object Detection Model
- YOLOv8 GPU: Unlocking Power with GPUs
- YOLOv8 Dataset Format: Mastering YOLOv8 Dataset Preparation
- YOLOv8 PyTorch Version: Speed and Accuracy in Your PyTorch Projects
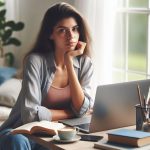
I’m Jane Austen, a skilled content writer with the ability to simplify any complex topic. I focus on delivering valuable tips and strategies throughout my articles.